Easy 2 mins tutorial on FOR loop to print array in TypeScript
Learn how to use for loop to print array in TypeScript
In this tutorial, we will learn to use for loop in TypeScript to print array items and all the elements in the array. If you are not aware of creating an array or publishing it, kindly follow the links given below.
Basic of TypeScript: First program in TypeScript
Array in TypeScript: Creating and printing array in TypeScript
To print an array using the for loop, first, you should understand the syntax of FOR loop in TypeScript. Following this, we will see how to use the FOR loop to print an entire array or print a particular element in the array.
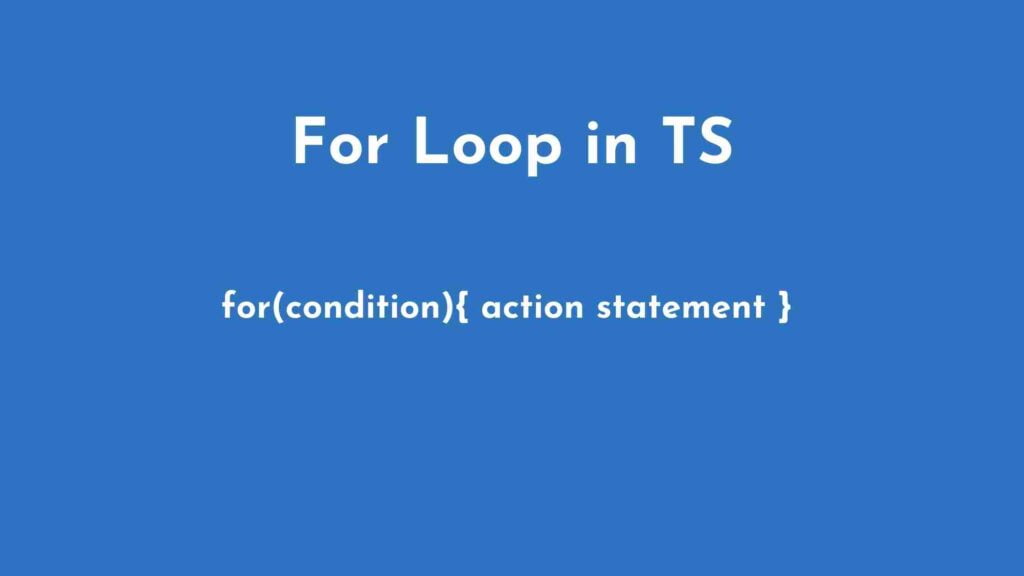
FOR loop Syntax
for(condition){
action statement
}
To demonstrate how to create a FOR loop to print an array, first, we need to create an array. Here we are creating an array of students.
let student_List = [
{name:'John', id:12345, class:'Bio'},
{name:'Peter', id:12346, class:'Bio'},
{name:'Adam', id:12347, class:'IT'}
]
The above statement creates the student list with NAME, ID and CLASS paraments. To print it normally, we can use the console statement.
console.log(student_List[1])
The above state will print the first student details, along with all the paraments. Now we can see, how to print the array with FOR loop for the same array student_List
for(let x of student_List){
console.log(x)
}
The above statement will produce a result with all the records and all the details as follows:
{name:’John’, id:12345, class:’Bio’},
{name:’Peter’, id:12346, class:’Bio’},
{name:’Adam’, id:12347, class:’IT’}
To print a particular parameter, i.e., NAME parameter from the above array, use the following code:
for(let x of student_List){
console.log(x.name)
}
The above statement will produce a result with all the records but with only the NAME parameter. The result is as follows:
John
Peter
Adam
Now that you have learnt about FOR loop to print array. You can also check our tutorial on Interfaces In TypeScript. Check our Angular with Angular Material Training from Ampersand Academy