Is your website contact form spammed by bots? Learn to implement PHP Contact Form with Google Recaptcha.
Hello everyone! By now, you would have gotten irritated with spam coming to your inbox from your contact form. Here is a quick tutorial on avoiding bot spams from your contact form by implementing Google Recaptcha version 2.
Contact form creation
- Name
- Mobile
- Message
In this tutorial, we will guide you on how to create a simple contact form using Bootstrap 5 with the following fields:
We will use the post method. First, we will have to create an HTML file using Bootstrap 5. We can use the Bootstrap 5 started template for designing the contact form using the following link
https://getbootstrap.com/docs/5.0/getting-started/introduction/
Create a file contact.html using any of the Text Editors
Paste the started template in the contact.html file.
Create fields based on your design requirement, or you can copy our code for design layouts. Also, embed the g-Recaptcha class in a division just above the submit button.
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-+0n0xVW2eSR5OomGNYDnhzAbDsOXxcvSN1TPprVMTNDbiYZCxYbOOl7+AMvyTG2x" crossorigin="anonymous">
<title>Contact Form with PHP, Bootstrap, Recaptcha</title>
<script src="https://google.com/recaptcha/api.js"></script>
<style>
.margintop {
margin-top: 25px;
margin-bottom: 25px
}
body {}
.card {
-webkit-box-shadow: 5px 5px 5px 0px rgba(50, 50, 50, 0.6);
-moz-box-shadow: 5px 5px 5px 0px rgba(50, 50, 50, 0.6);
box-shadow: 5px 5px 5px 0px rgba(50, 50, 50, 0.6);
}
.g-recaptcha{
margin-top: 25px;
margin-left: auto;
margin-right: auto
}
</style>
</head>
<body>
<div class="row container margintop">
<div class="col-md-4 offset-md-5 margintop">
<form method="post" action="contact.php">
<div class="card" style="padding: 10px;background-image: linear-gradient( 109.6deg, rgba(116,18,203,1) 11.2%, rgba(230,46,131,1) 91.2% ); ">
<h1 class="margintop" style="text-align:center; color: white">Contact Form</h1>
<div class="form-floating mb-3">
<input type="text" class="form-control" id="Name" name="name" placeholder="John Doe">
<label for="name">Name</label>
</div>
<div class="form-floating mb-3">
<input type="mobile" class="form-control" id="mobile" name="mobile" placeholder="+91 9876543210">
<label for="mobile">Mobile</label>
</div>
<div class="form-floating mb-3">
<input type="email" class="form-control" id="email" name="email" placeholder="johndoe@gmail.com">
<label for="email">Email</label>
</div>
<div class="form-floating">
<textarea class="form-control" placeholder="Leave a comment here" id="message" name="message" style="height: 100px"></textarea>
<label for="message">Message</label>
</div>
<div class="g-recaptcha" data-sitekey="Include Site Key from Google Recaptcha"></div>
<p class="margintop" align="center">
<button type="submit" class="btn btn-warning">Submit</button>
</p>
</div>
</form>
</div>
</div>
<!-- Optional JavaScript; choose one of the two! -->
<!-- Option 1: Bootstrap Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.1/dist/js/bootstrap.bundle.min.js" integrity="sha384-gtEjrD/SeCtmISkJkNUaaKMoLD0//ElJ19smozuHV6z3Iehds+3Ulb9Bn9Plx0x4" crossorigin="anonymous"></script>
<!-- Option 2: Separate Popper and Bootstrap JS -->
<!--
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.9.2/dist/umd/popper.min.js" integrity="sha384-IQsoLXl5PILFhosVNubq5LC7Qb9DXgDA9i+tQ8Zj3iwWAwPtgFTxbJ8NT4GN1R8p" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.1/dist/js/bootstrap.min.js" integrity="sha384-Atwg2Pkwv9vp0ygtn1JAojH0nYbwNJLPhwyoVbhoPwBhjQPR5VtM2+xf0Uwh9KtT" crossorigin="anonymous"></script>
-->
</body>
</html>
Creating PHP form to Capture and Validate Data
Create the PHP file called contact.php
Create variables name, email, mobile, message and other variables as required to send the email.
<?php
$name = $_POST['name'];
$email = $_POST['email'];
$mobile = $_POST['mobile'];
$message = $_POST['message'];
$email_from ='Your Name';
$email_subject = "New Message from Website Name";
$email_body = "Name: $name.\n".
"Email: $email.\n".
"Mobile: $mobile.\n".
"Message: $message.\n";
$to = "info@yourdomain.com";
$headers = "From: $email_from \r \n";
$headers .="Reply-To: $email \r \n";
$secretKey = "Google Recaptcha V2 Secret";
$responseKey = $_POST['g-recaptcha-response'];
$userIP = $_SERVER['REMOTE_ADDR'];
$url="https://www.google.com/recaptcha/api/siteverify?secret=$secretKey&response=$responseKey&remoteip=$userIP";
$response = file_get_contents($url);
$response = json_decode($response);
if ($response->success){
mail($to,$email_subject,$email_body,$headers);
echo '<script>alert("Thank you! We will contact Soon"); window.location = "contact.html";
</script>';
}
?>
Getting Recaptcha Site Key & Secret Key
Go to the Google Recaptcha Website using the link: https://www.google.com/recaptcha/admin/create
Enter details and select Recaptcha V2 since Google Recaptcha without checkbox doesn’t serve the purpose.
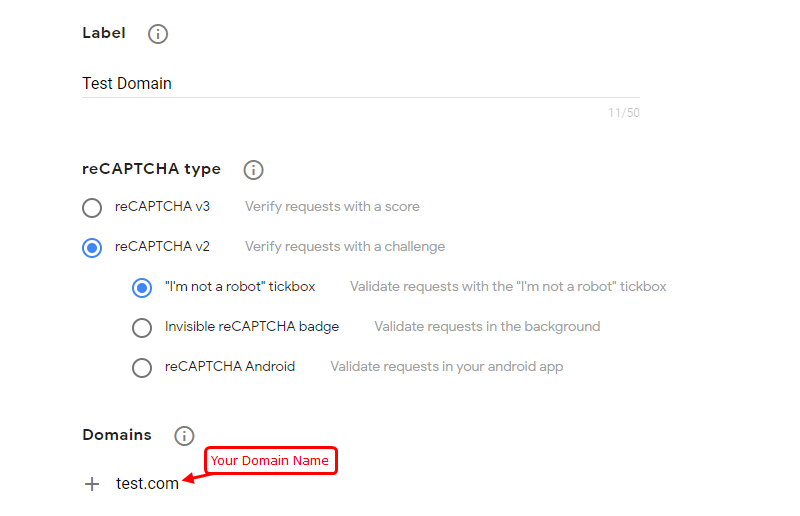
Copy the Recaptcha site key and paste it inside the Recaptcha Button.
Now copy the secret key and paste it inside the secret key variable.
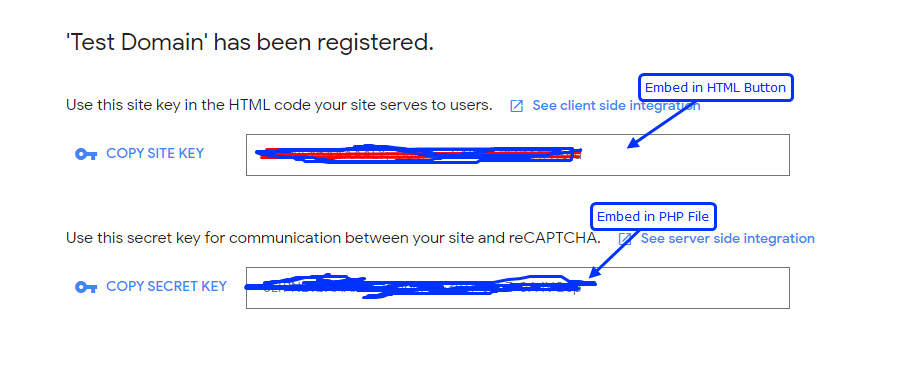
Include form tag before the start of the field. Name your PHP form, and action tag, call the contact.php file.
If the website user checks the Recaptcha, it will pass various parameters to the Google Recaptcha API. The PHP form will send all details filled in the contact form to the email if API returns success.
Now we have successfully integrated the Recaptcha V2.
By successfully integrating Google Recaptcha V2 on your contact form you can prevent spambots in your website contact form. You can feel free to use this code to create a contact form with Google Recaptcha on your websites. Check the tutorial on Materialize CSS